SQL Server Function for concatenating strings
Displaying well formatted text
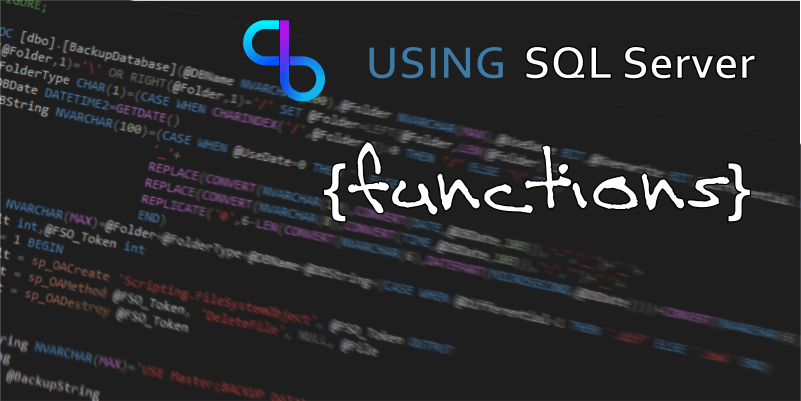
This function was born out of the need to display an address in a well formatted string. When working with this sort of data, there are often blanks, NULL values and sometimes separated text within the data fields. Standard concatenation of the columns led to double delimiters with blank spaces or commas at the end.
With this in mind we needed a way of separating out the data, excluding the blanks, trimming white space, and removing the trailing data. There are various ways of using standard COALESCE alone, but that didn't quite cut it with the blanks.
We used our tried and tested TextToRows function to split and sort the text, then wrote the text values back into a new string. The benefit of this is that the data is re-usable with delimiters of your choice for both input and output.
SQL
ALTER FUNCTION dbo.TextConc(@Text NVARCHAR(MAX),@Delim CHAR(1),@Sep CHAR(2)) RETURNS NVARCHAR(MAX) WITH SCHEMABINDING AS BEGINDECLARE @Str NVARCHAR(MAX)SELECT @Str=COALESCE(@Str+@Sep,'')+REPLACE(WordStr,@Delim,'')FROM dbo.TextToRows(@Delim,@Text)WHERE WordStr<>''RETURN REPLACE(@Str,@Sep+@Sep,@Sep)ENDGO
Test Data
For testing purposes we can create a temporary table and store a couple of addresses. Combine the select statement with function and you can see how it returns a crisply formatted data set that is perfect for display purposes.
SQL
DECLARE @Address TABLE(AddressName NVARCHAR(100),AddressL1 NVARCHAR(100),AddressL2 NVARCHAR(100),AddressL3 NVARCHAR(100),AddressCity NVARCHAR(100),AddressCounty NVARCHAR(100),AddressCountry NVARCHAR(100),AddressPostCode NVARCHAR(100))INSERT INTO @AddressSELECT 'Mountain View','1600 Amphitheatre Parkway',NULL,NULL,'Mountain View','California','United States','94043'INSERT INTO @AddressSELECT 'London','1-13 St Giles High St',NULL,NULL,'London','London','United Kingdom','WC2H 8LG'SELECT dbo.TextConc(ISNULL(AddressName,'')+','+ISNULL(AddressL1,'')+','+ISNULL(AddressL2,'')+','+ISNULL(AddressL3,'')+','+ISNULL(AddressCity,'')+','+ISNULL(AddressCounty,'')+','+ISNULL(AddressCountry,'')+','+ISNULL(AddressPostCode,''),',',', ')FROM @Address
Results
Persist the data in the table
As we created the data with SCHEMABINDING, we can also append it to a table as a computed column.
Note, when adding these, it can impact query performance, so we generally try to keep the data persisted, this actually stores the data in the table, and it is only calculated on insert/update transactions.
SQL
CREATE TABLE TestAddress(AddressName NVARCHAR(100),AddressL1 NVARCHAR(100),AddressL2 NVARCHAR(100),AddressL3 NVARCHAR(100),AddressCity NVARCHAR(100),AddressCounty NVARCHAR(100),AddressCountry NVARCHAR(100),AddressPostCode NVARCHAR(100),AddressDisplay as dbo.TextConc(ISNULL(AddressName,'')+','+ISNULL(AddressL1,'')+','+ISNULL(AddressL2,'')+','+ISNULL(AddressL3,'')+','+ISNULL(AddressCity,'')+','+ISNULL(AddressCounty,'')+','+ISNULL(AddressCountry,'')+','+ISNULL(AddressPostCode,''),',',', ') PERSISTED)INSERT INTO TestAddressSELECT 'Mountain View','1600 Amphitheatre Parkway',NULL,NULL,'Mountain View','California','United States','94043'INSERT INTO TestAddressSELECT 'London','1-13 St Giles High St',NULL,NULL,'London','London','United Kingdom','WC2H 8LG'SELECT * FROM TestAddressDROP TABLE TestAddress